173. TODO
Media
Video
Coming soon.
Podcast
Coming soon.
WG21 Meeting, St Louis, 2024
C++26 will have P2300 std::execution
!
No progress on pattern matching, it seems…
Reflection in C26: the renaissance of C
CppCast 383: Safe, Borrow-Checked, C++
How often do you create memory leak or segfault bugs with modern C++?
Memory leaks - pretty much never. RAII takes care of those way too well for them to happen. The only time I may make them is if I have a resource that I simply don’t know I have to cleanup. Segfaults - I do sometimes make them, particularly during development. They are much more likely when I interface with very low level code (e.g. OS APIs or other low-level libraries), which tends to have many pointers and/or poor type safety. In high level code I make them much less commonly. Overall, I personally don’t find memory management in C++ to be too troublesome. ↑
Falsehoods programmers believe about undefined behavior
The moment your program contains UB, all bets are off.
Even if it’s just one little UB.
Even if it’s never executed.
Even if you don’t know it’s there at all.
Thinking of using this next time when I have to prove that my UB fix is actually necessary.
Boost: good or bad?
From Reddit:
Annoyed with Overuse of Boost in C++ Discussions
For instance, I was recently exploring Interprocess, only to find out I needed Boost.Date, which led me to implement it myself rather than dealing with the extra dependency.
Ah yes, a date library, famously easy to implement. (OK, they used a std::chrono wrapper, but still.)
And in your lack of experience, you committed a cardinal sin of programming. NEVER write your own datetime implementation unless you are prepared to dedicate a team to maintain it. Time zones are impossible, leap seconds will screw you, and numerous other pitfalls await. It’s almost always a terrible idea. Use a library that gets tons of real world use and debugging. ↑
Boost is now modular you don’t need to build the full Boost Set of libraries + with vcpkg it is straightforward : I don’t understand why people try to reimplement sub parts of Boost in worse way. Sometime you can find better libraries than the Boost ones and that perfect, but imo Boost is the first to be tried. ↑
We here use boost extensively, both server and embedded. Honestly I don’t know what all the fuss is about. It’s excellent code, well maintained by top folks in the c++ world. If you don’t like it, don’t use it. But don’t whine when other people do. ↑
Alternatives to Boost
https://www.reddit.com/r/cpp/comments/1cpwyqj/whats_your_favorite_boost_alternative/
Are We Modules Yet?
The C++20 Naughty and Nice List for Game Devs
Learn Modern C++
A self-study C++20 course by Richard Spencer
Lambdas in C++23
argparse 2.9 released
Stronk - a strong type and unit library
Can you link 2 binaries compiled with 2 different C++ compilers?
New C++23 features I’m excited about, by Tomasz Wisniewski
Revisiting Turbo C++
See also: The IDEs we had 30 years ago… and we lost, discussed on HN and Lobsters
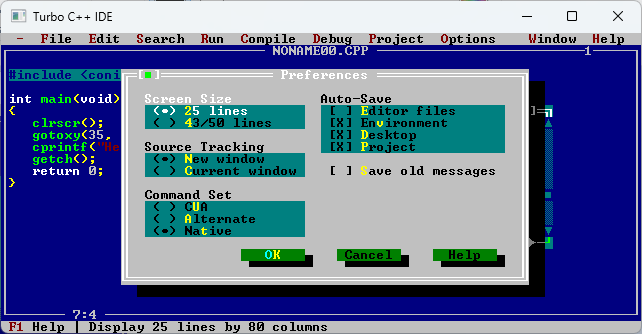
A visual history of Visual C++
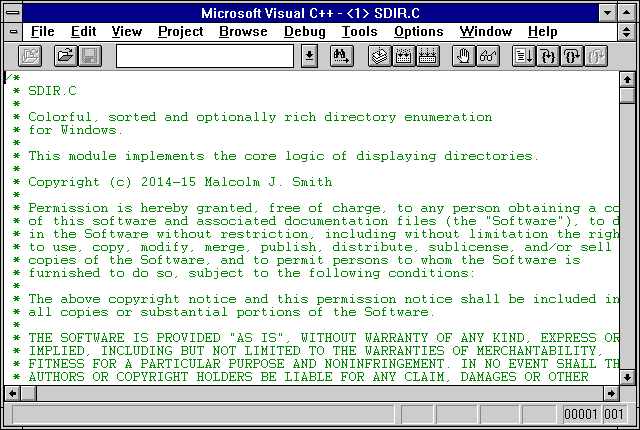
A Year of C++ Improvements in Visual Studio, VS Code, and vcpkg
Book: C++ Initialization Story, by Bartłomiej Filipek
See also: I HAVE NO CONSTRUCTOR, AND I MUST INITIALIZE, by Evan Girardin (Nice website banner, strong PDP-11 vibes). Discussion on HN.
Speeding up C++ build times
Blender forum: Speeding up C++ builds
Working With Jumbo/Unity Builds (Single Translation Unit)
https://austinmorlan.com/posts/unity_jumbo_build/ by Austin Morlan
Figma
Subspace
Accidentally hiding base virtual functions
#include <iostream>
struct B
{
virtual ~B() = default;
virtual void foo() { std::cout << "B::foo()\n"; }
virtual void foo(int) { std::cout << "B::foo(int)\n"; }
};
struct D1 : B
{
void foo() override { std::cout << "I::foo()\n"; }
// ^ MSVC warning 4266: no override for foo(int); function is hidden
};
struct D2 final : D1
{
void foo() override { std::cout << "D::foo()\n"; }
void foo(int) override { std::cout << "D::foo(int)\n"; }
};
int main(int /*argc*/, char** /*argv*/)
{
B b;
b.foo();
b.foo(0);
D1 i;
i.foo();
i.foo(0); // Does not compile: base function is hidden
i.B::foo(0); // Call base version
D2 d;
d.foo();
d.foo(0);
return 0;
}
Mastodon: preventing implicit conversions
https://mastodon.social/@ohunt/112294336934673348
Oliver Hunt:
I was today years old when I discovered you can stop implicit bool→int conversion in APIs where it causes problems by doing
int foo(int) {...}
int foo(bool) = delete;
Despite knowing this is a valid syntax it never occurred to me you could use it this way, and I did not realize you can do this for free functions O_o
Why CMake sucks
Follow-ups by Tomasz Wisniewski:
See also:
ppstep Interactive Macro Debugger
Mastodon: Debugging
https://octodon.social/@splitbrain/112086905723468442
I really think debugging should be taught in school. Not for any programming language. Kids should learn how to systematically approach a problem, gather diagnostic, follow cause and effect and how to communicate the problem to others. Regardless if this is computer stuff, plumbing or social sciences.
Bluesky
AI company: we trained this dog to talk. It doesn’t actually understand language, but it kinda sounds like it’s having a conversation by mimicking the sound of human speech.
CEO: awesome, I’ve fired my entire staff, how quickly can it start diagnosing medical disorders
Slop
Remote workers
devopscats via Mastodon: https://toot.cat/@devopscats/112566801377839795
Behind every remote worker is a cat that hasn’t signed an NDA and will sell all the secrets for a piece of sashimi.
Quote
https://mastodon.social/@programming_quotes/112572400857683147
One of the best programming skills you can have is knowing when to walk away for awhile.
Mastodon: Full-stack developer
https://digipres.club/@foone/112412593654054471
I’m a "full stack developer", in that my stack is full and if you try to push any more tasks on me I’m gonna overflow it and start corrupting my own memory