Meeting 20 June 2019
CPPP19 Trip Reports
Hello World with reflection
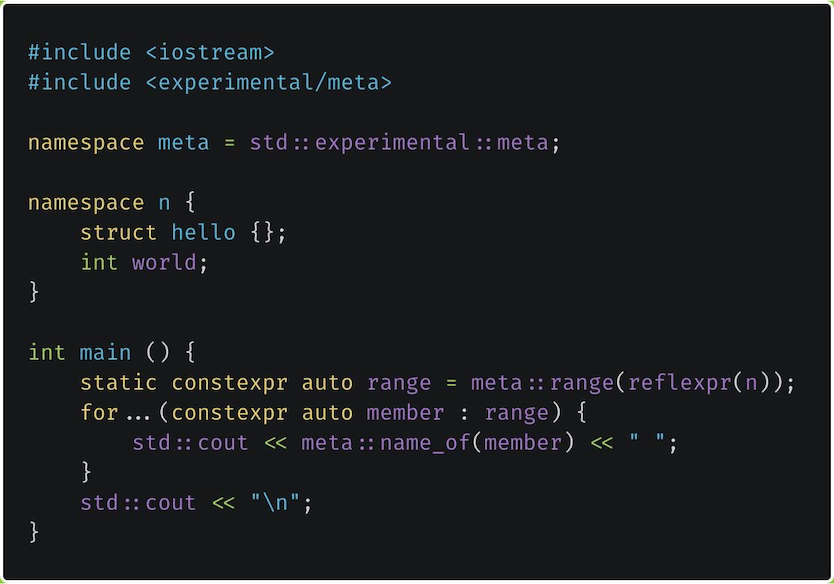
Heterogeneous Lookup in Ordered Containers in C++14
https://www.bfilipek.com/2019/05/heterogeneous-lookup-cpp14.html
https://www.reddit.com/r/cpp/comments/btrfnd/heterogeneous_lookup_in_ordered_containers_c14/
Professional, zero-cost setup for C++ projects
Part 1: https://awfulcode.io/2019/04/13/professional-zero-cost-setup-for-c-projects-part-1-of-n/
Part 2: https://awfulcode.io/2019/04/26/professional-zero-cost-setup-for-c-projects-part-2-of-n/
Reddit: https://www.reddit.com/r/cpp/comments/bhqcjh/professional_zerocost_setup_for_c_projects_part_2/
Higher-order functions
Meeting C++ 2018: Björn Fahller - Higher Order Functions for ordinary developers
A higher-order function is a function that takes other functions as arguments or returns a function as result.
Take-away messages:
- Avoid using std::function as return type, use auto instead
- Capturing by reference when returning a lambda is dangerous
- Compose functions and give names to compositions
- Functional extensions to std::optional and std::expected remove the need for many conditionals
PacifiC++ 2018 - Titus Winters - C++ Past vs. Future
std::function const correctess bug
struct Callable { void operator()(){count++;} void operator()() const = delete; int count = 0; }; void f() { Callable counter; std::function<void(void)> f = counter; f(); const auto cf = f; cf(); // ??? }
PacifiC++ 2018 - Titus Winters - C++ Past vs. Future (cont.)
std::function requires copyable callables
void f() { std::unique_ptr<int> up; auto l=[up=std::move(up)](){}; std::function<void(void)> f=l; // Build fails }
PacifiC++ 2018 - Titus Winters - C++ Past vs. Future (cont.)
ODR violation
namespace libs { inline bool contains(std::string_view needle, std::string_view haystack) { assert(needle.size() <= haystack.size()); // !!! return haystack.find(needle) != std::string_view::npos; } }
C++ Developer Ecosystem
https://www.jetbrains.com/lp/devecosystem-2019/cpp/
https://www.reddit.com/r/cpp/comments/c21ft6/the_state_of_developer_ecosystem_2019_c/
Catch V2.9.0
https://github.com/catchorg/Catch2/releases/tag/v2.9.0
This release replaces the old benchmarking support with a new one, based on donated Nonius code.
Taskflow V2.2.0
Cpp-Taskflow is by far faster, more expressive, and easier for drop-in integration than existing parallel task programming libraries such as OpenMP Tasking and Intel TBB FlowGraph in handling complex parallel workloads.
https://github.com/cpp-taskflow/cpp-taskflow/releases/tag/v2.2.0 (MIT)
Docs: https://cpp-taskflow.github.io/cpp-taskflow/index.html
SFINAE and enable_if
https://eli.thegreenplace.net/2014/sfinae-and-enable_if/
https://www.reddit.com/r/cpp/comments/c1njko/sfinae_and_enable_if_eli_benderskys_website/
Boost out_ptr
https://thephd.github.io/vendor/future_cxx/papers/d1132.html
https://github.com/ThePhD/out_ptr
https://github.com/ThePhD/out_ptr/blob/master/docs/out_ptr.adoc
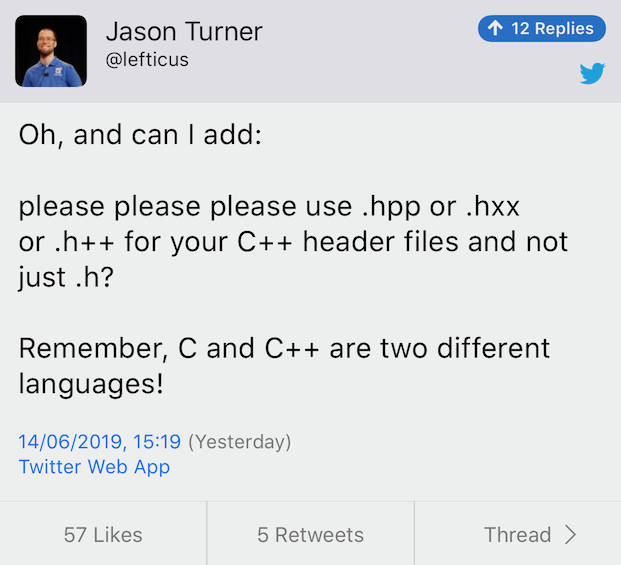
Quote
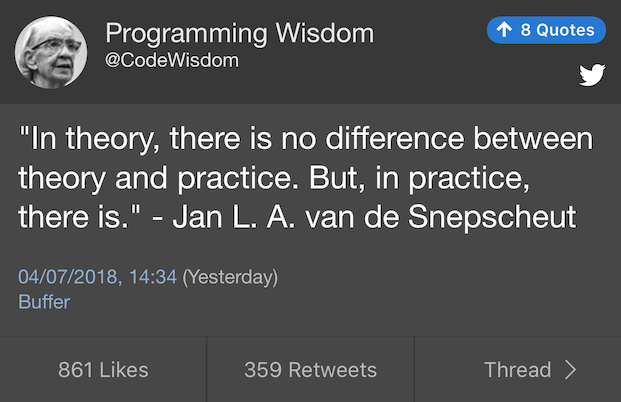